Coding
Challenge yourself, explore new concepts, and enhance your programming skills with our curated collection of programming problems. Let's embark on this journey of continuous learning and growth together!
Problems Registry
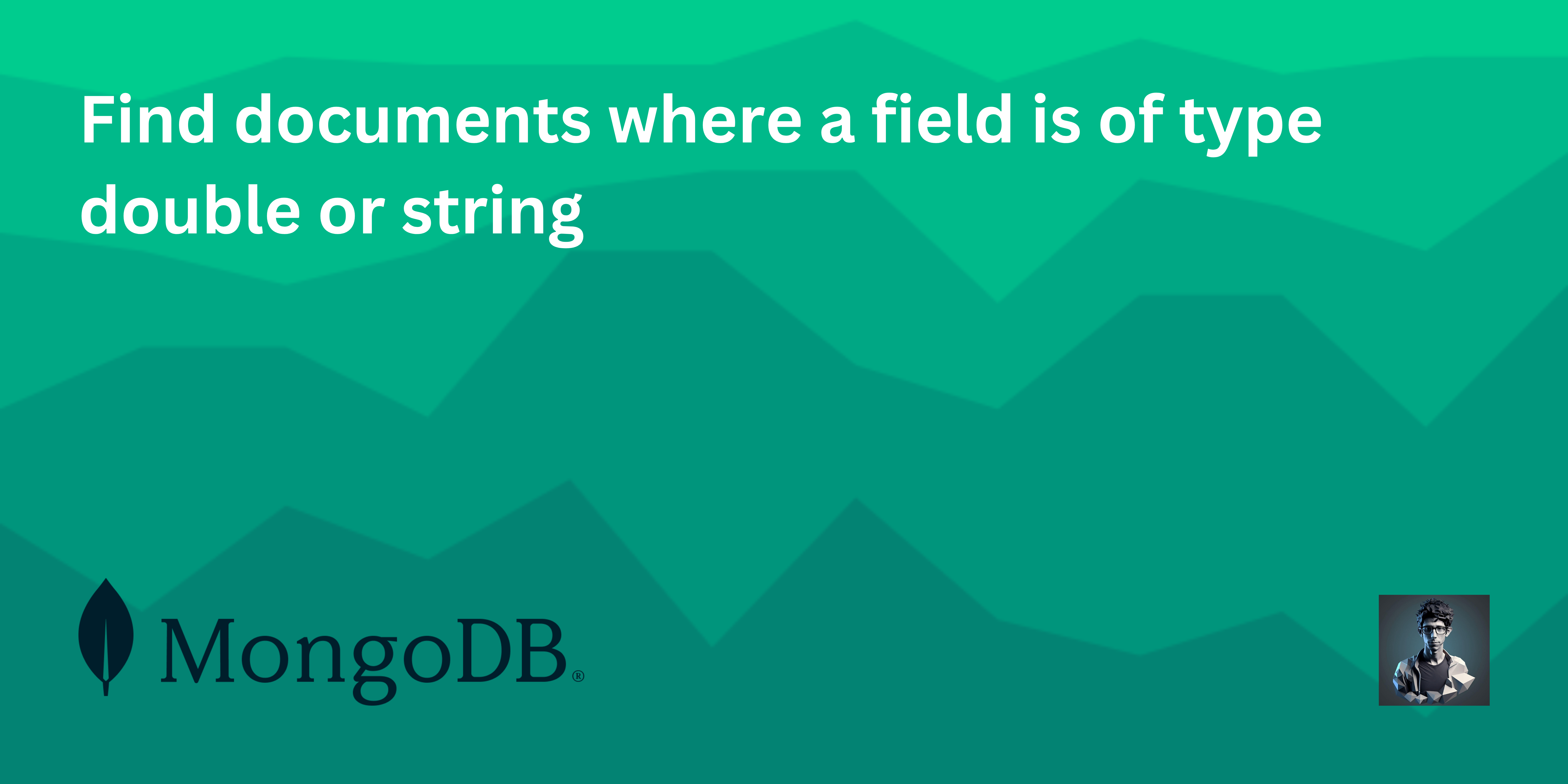
Find documents where a field is of type double or string
Write mongodb query to find document where field named 'age' have type double or string.
Solve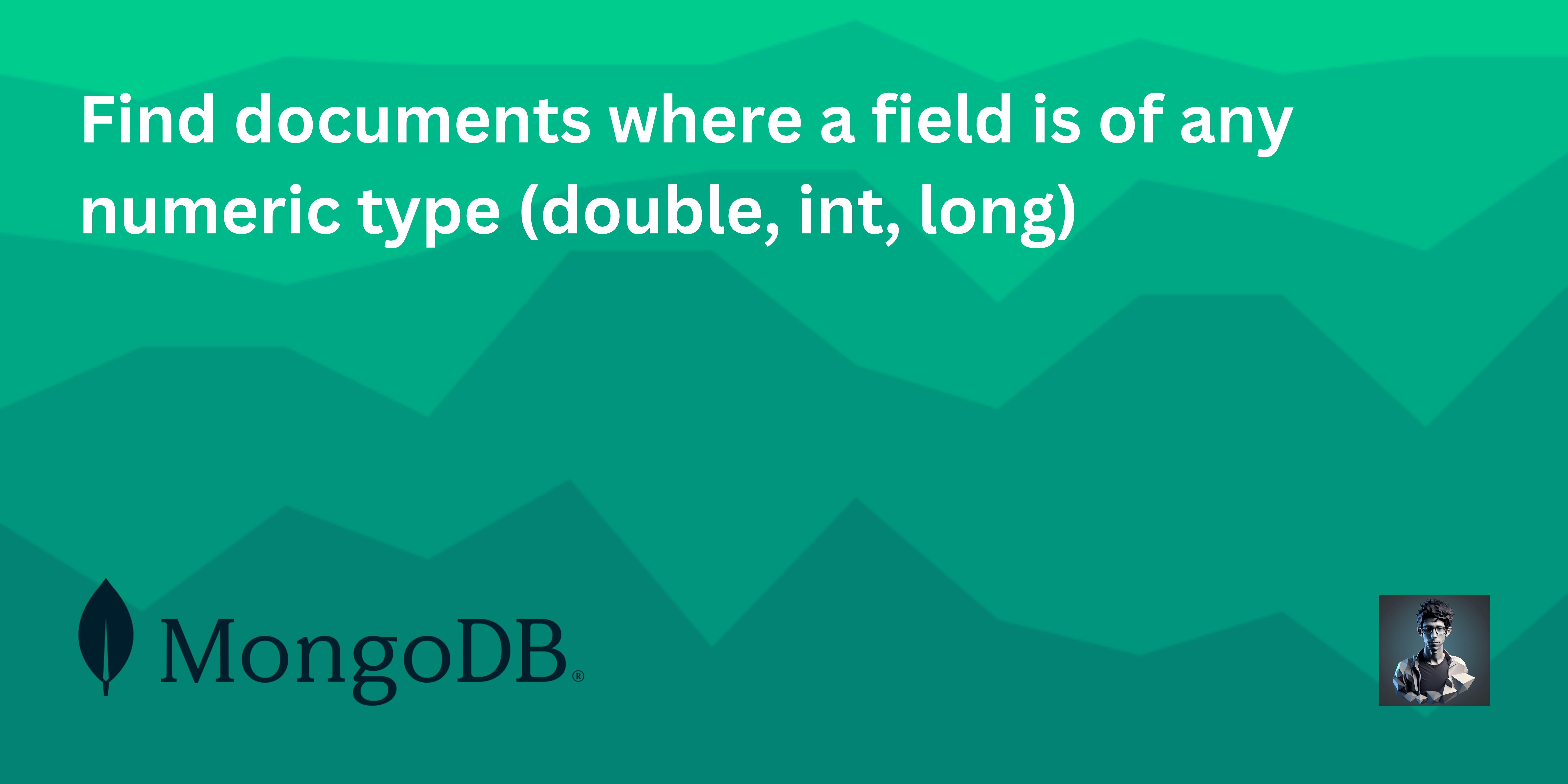
Find documents where a field is of any numeric type (double, int, long)
Write mongodb query to find document where field named 'age' have any numeric data type.
Solve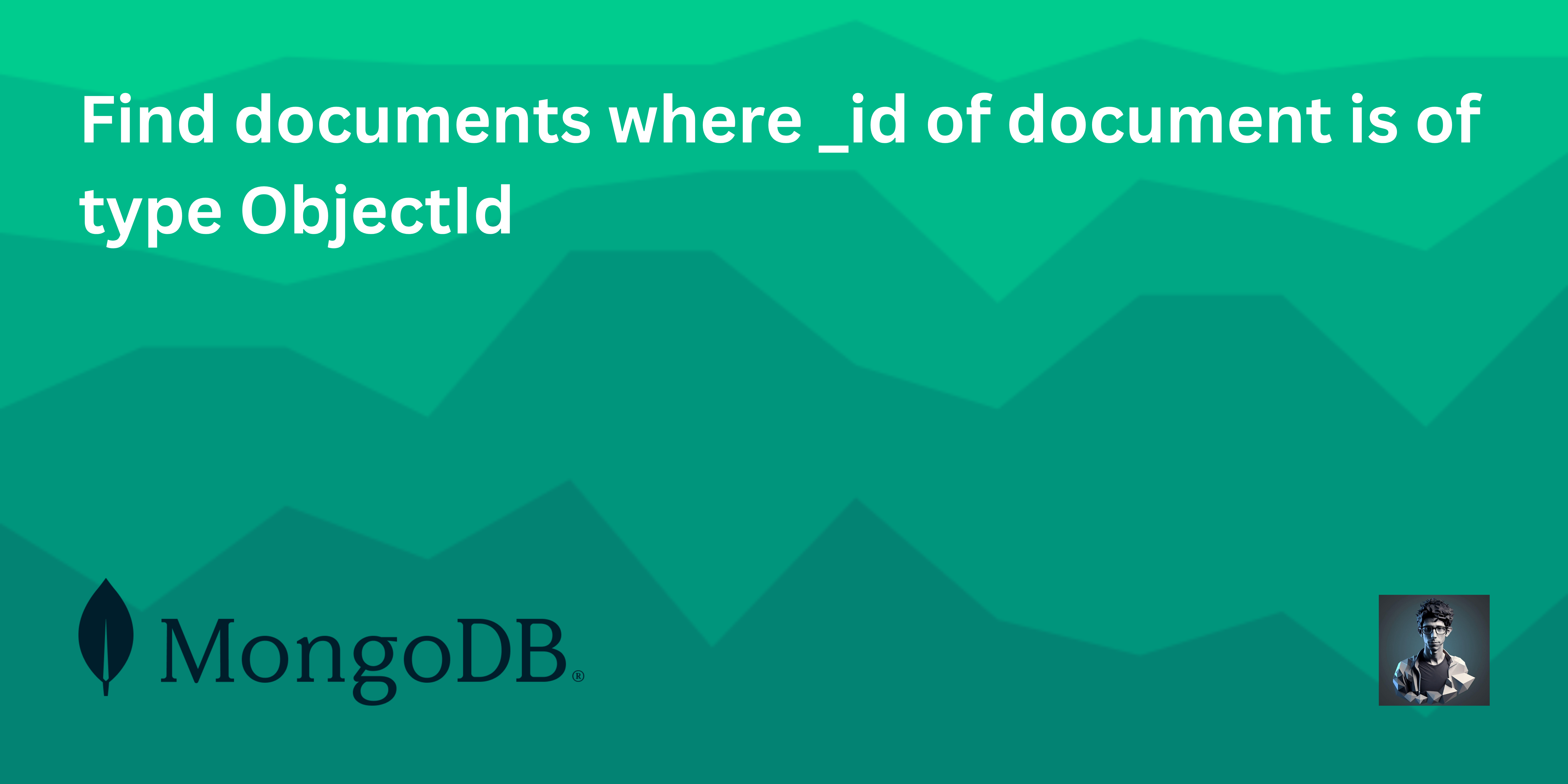
Find documents where _id of document is of type ObjectId
Write mongodb query to find documents where _id of document is of type ObjectId.
Solve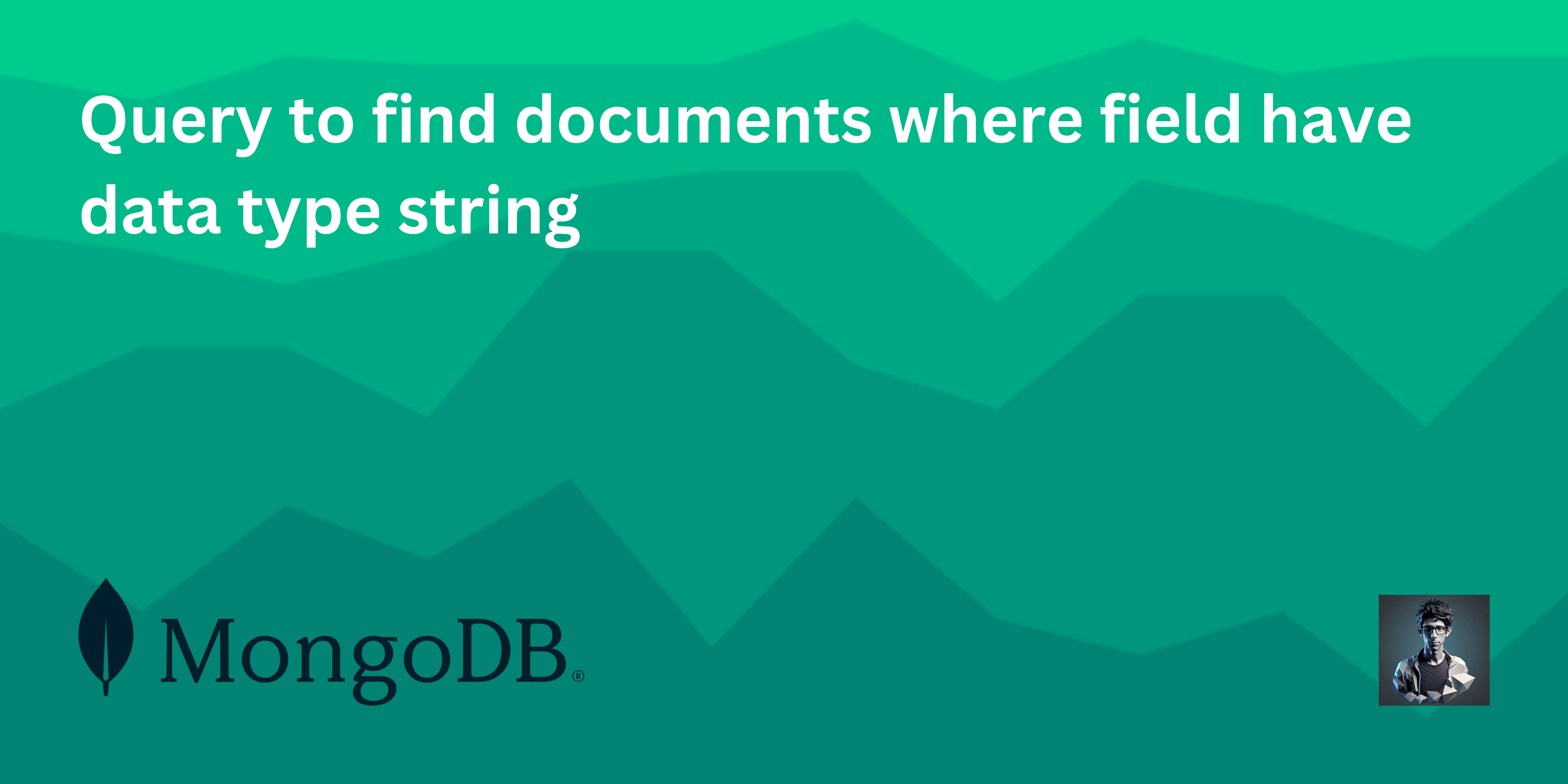
Query to find documents where field have data type string
Write mongodb query to find document where field named 'name' have data type String
Solve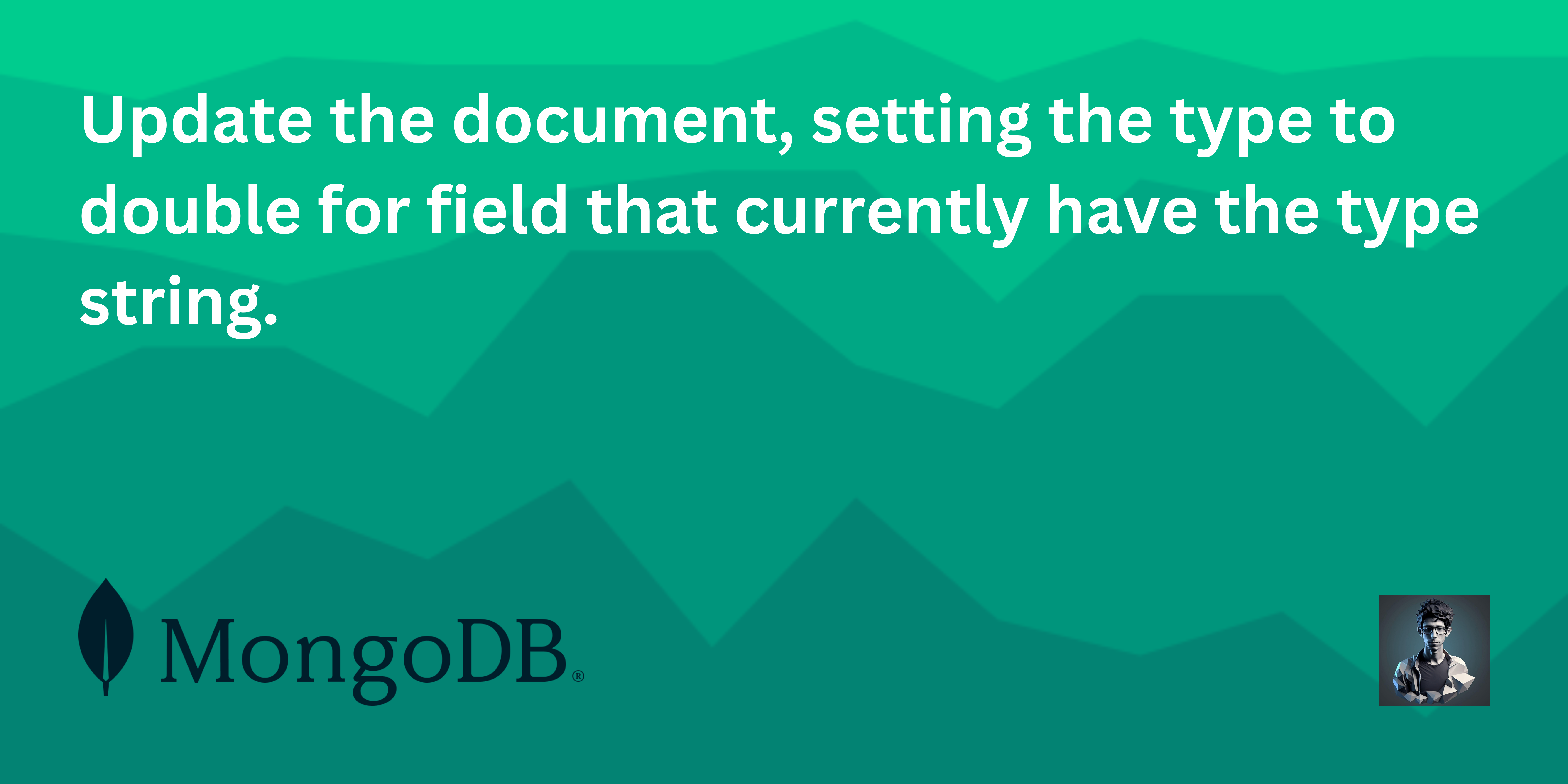
Update the document, setting the type to double for field that currently have the type string.
Write mongodb query to update the document, setting the type to double for field 'age' that currently have the type string.
Solve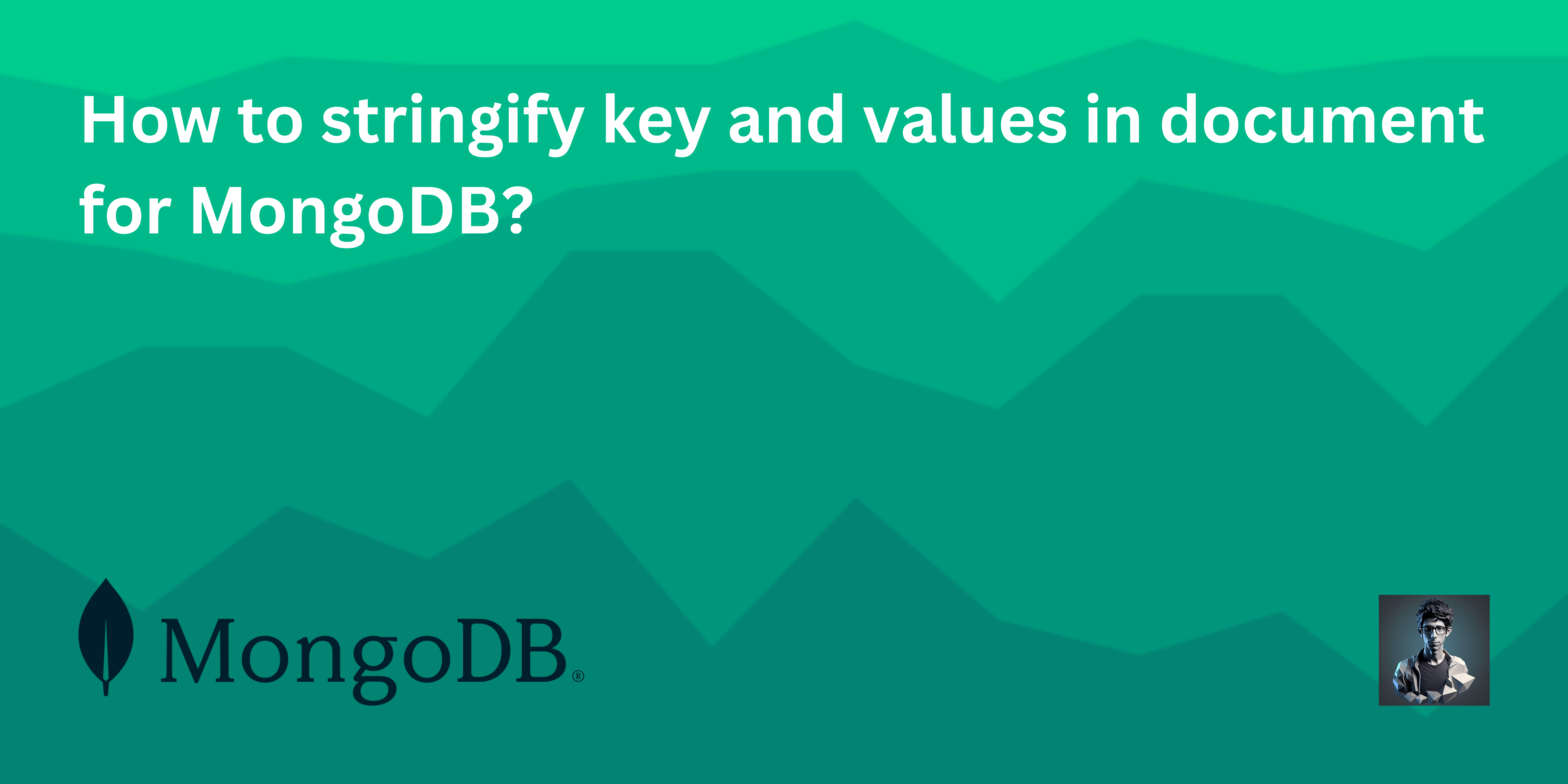
How to stringify key and values in document for MongoDB?
We have following collection movies, now stringify the document in collection where title is Inception
Solve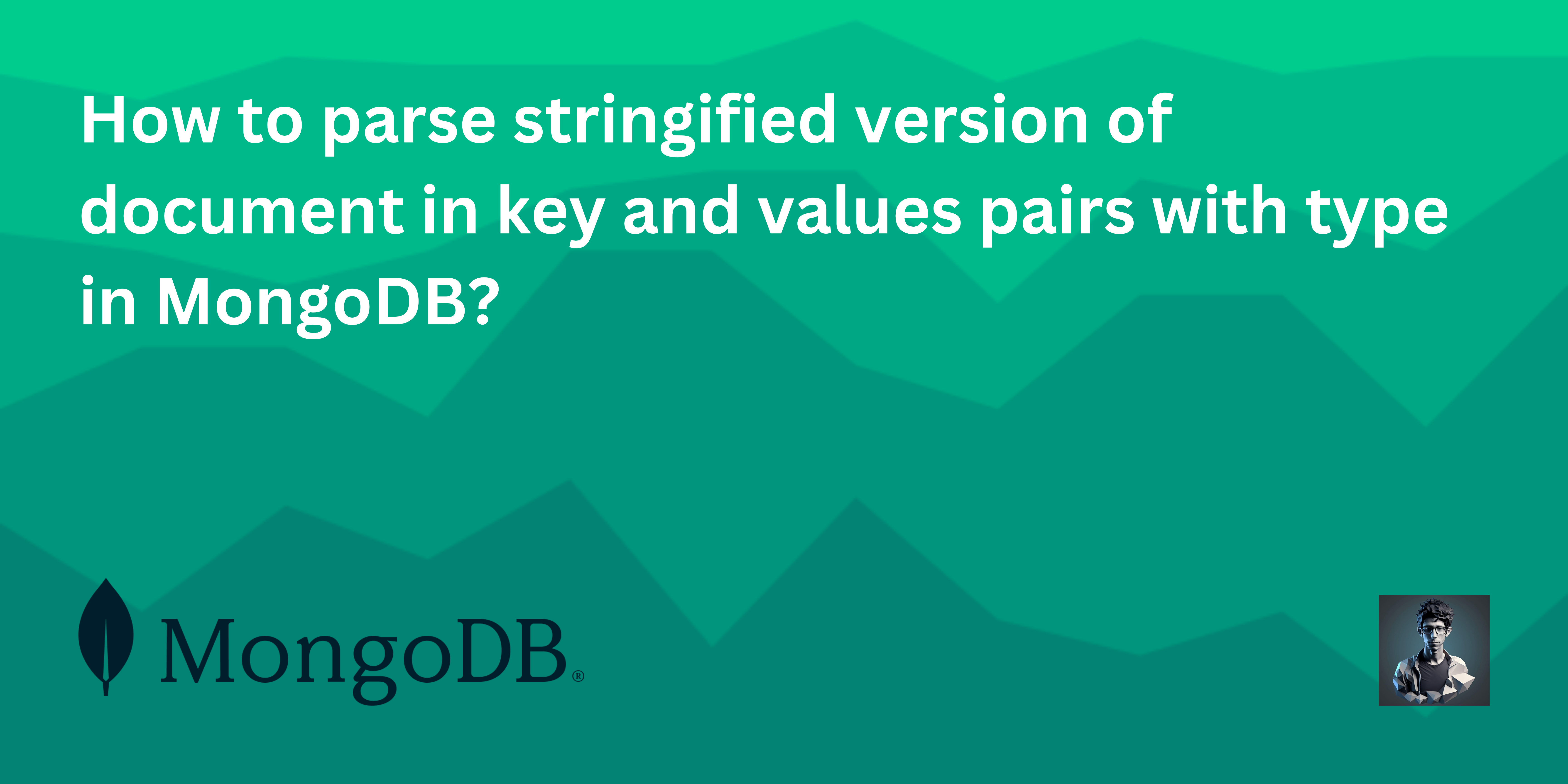
How to parse stringified version of document in key and values pairs with type in MongoDB?
We have following JSON object in stringified format. Convert that into document i.e. in key value pair
Solve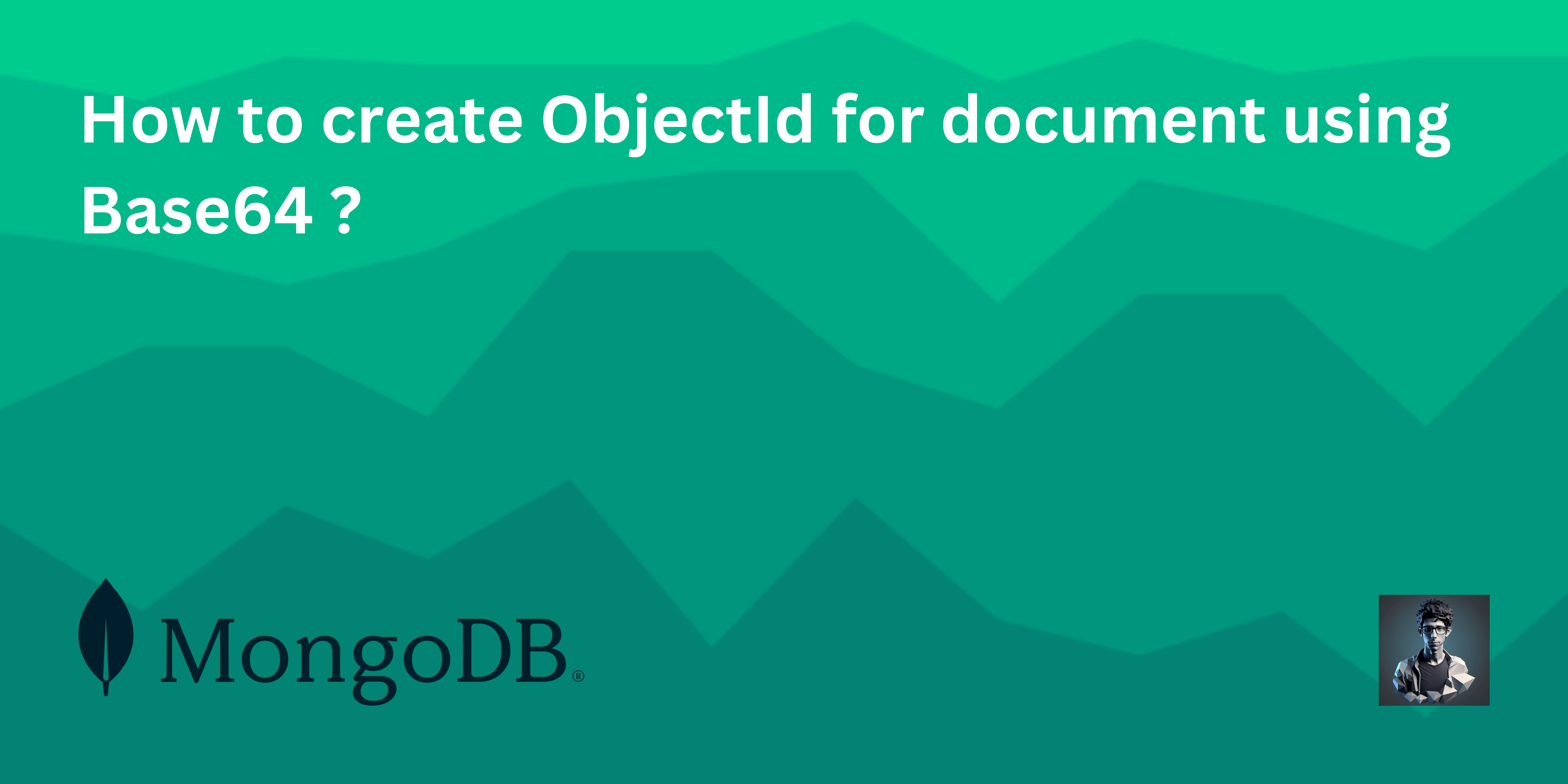
How to create ObjectId for document using Base64 ?
Write mongoDB query for inserting document with ObjectId created from base64 text.
Solve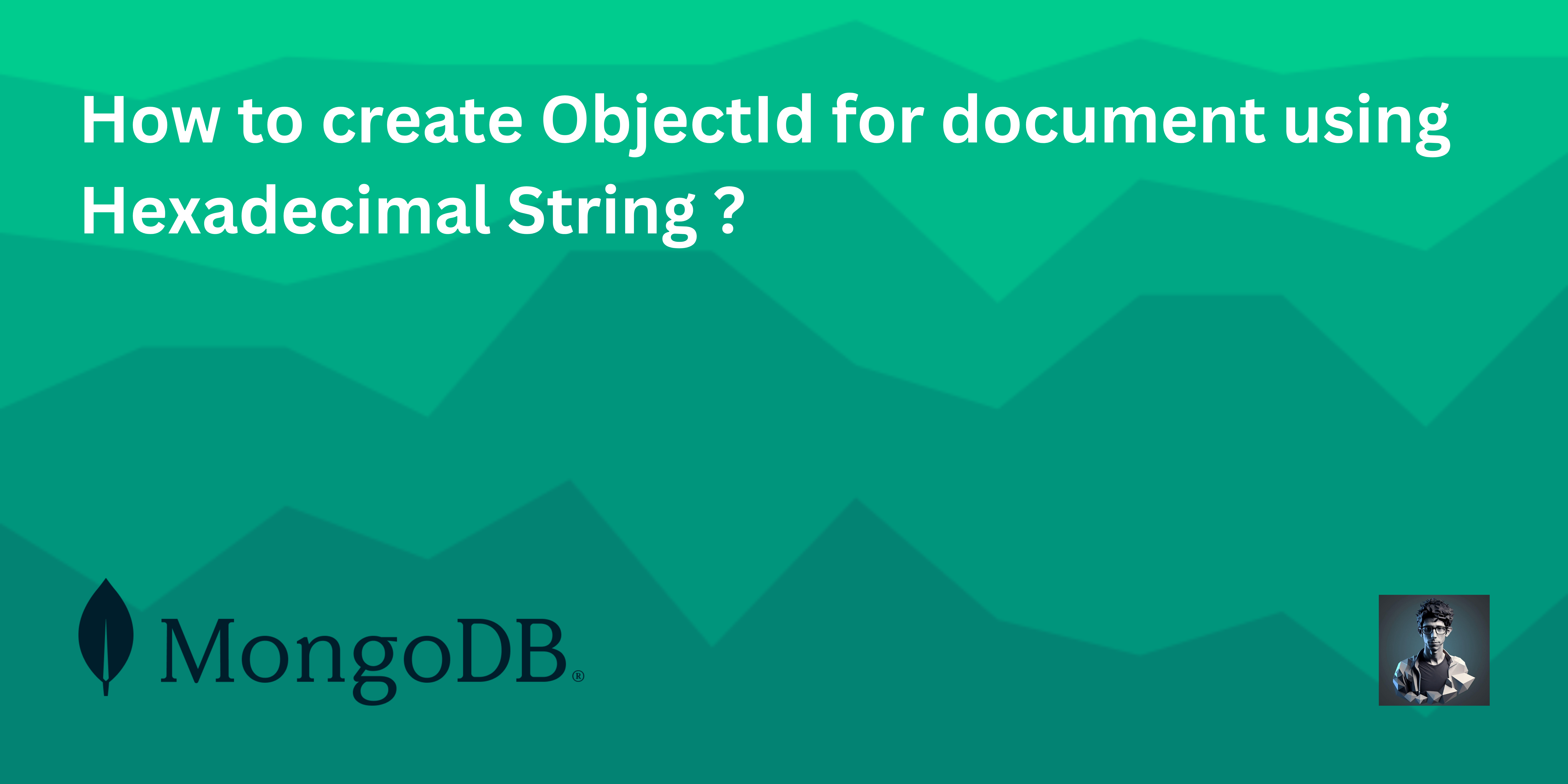
How to create ObjectId for document using Hexadecimal String ?
Write mongoDB query for inserting document with ObjectId created from hexadecimal string.
Solve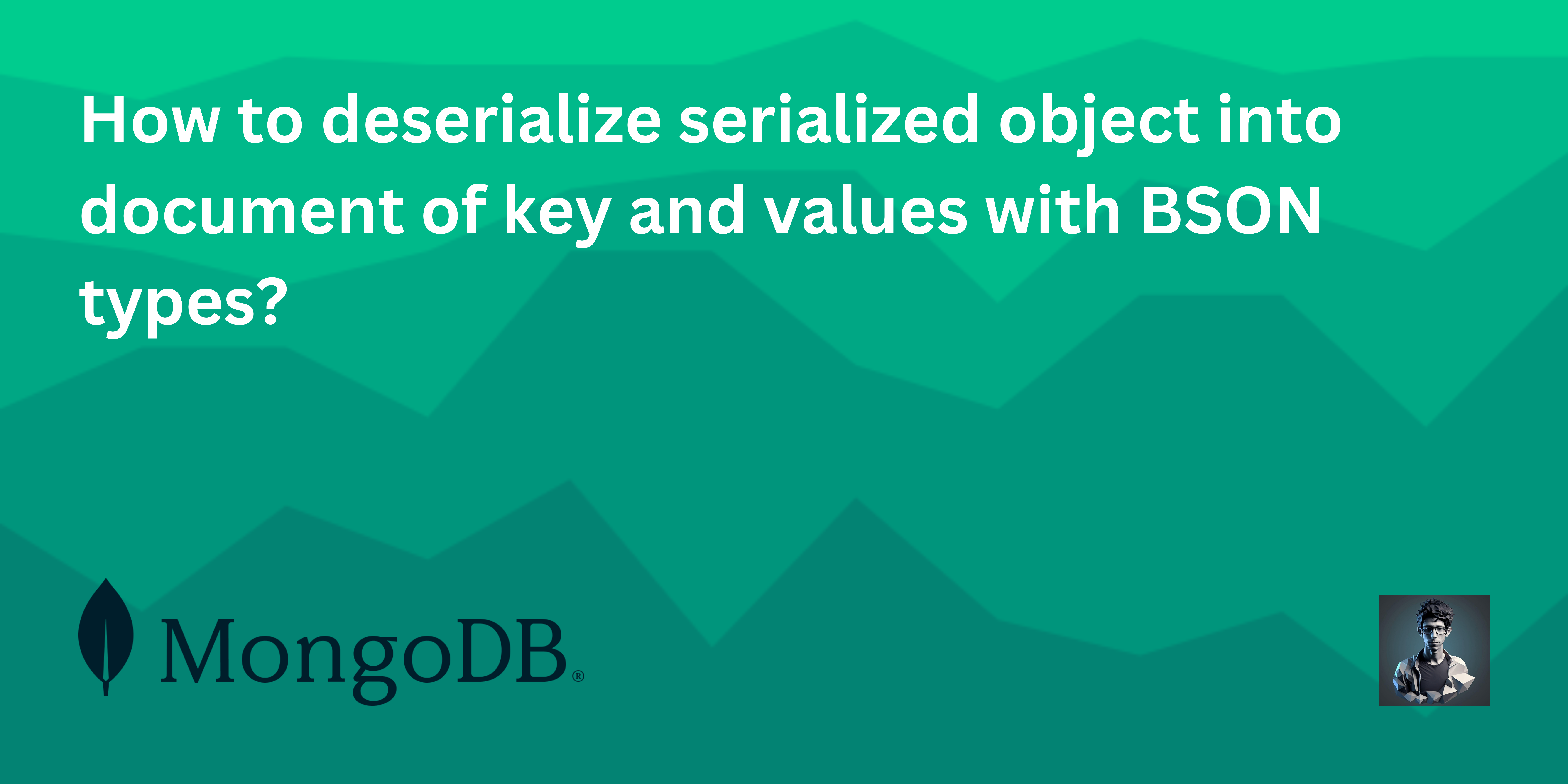
How to deserialize serialized object into document of key and values with BSON types?
We have following collection movies, and we created one variable serializedData which contains the serialized data for document where name is Lex. Now we have to deserialize it again in document of key and values.
Solve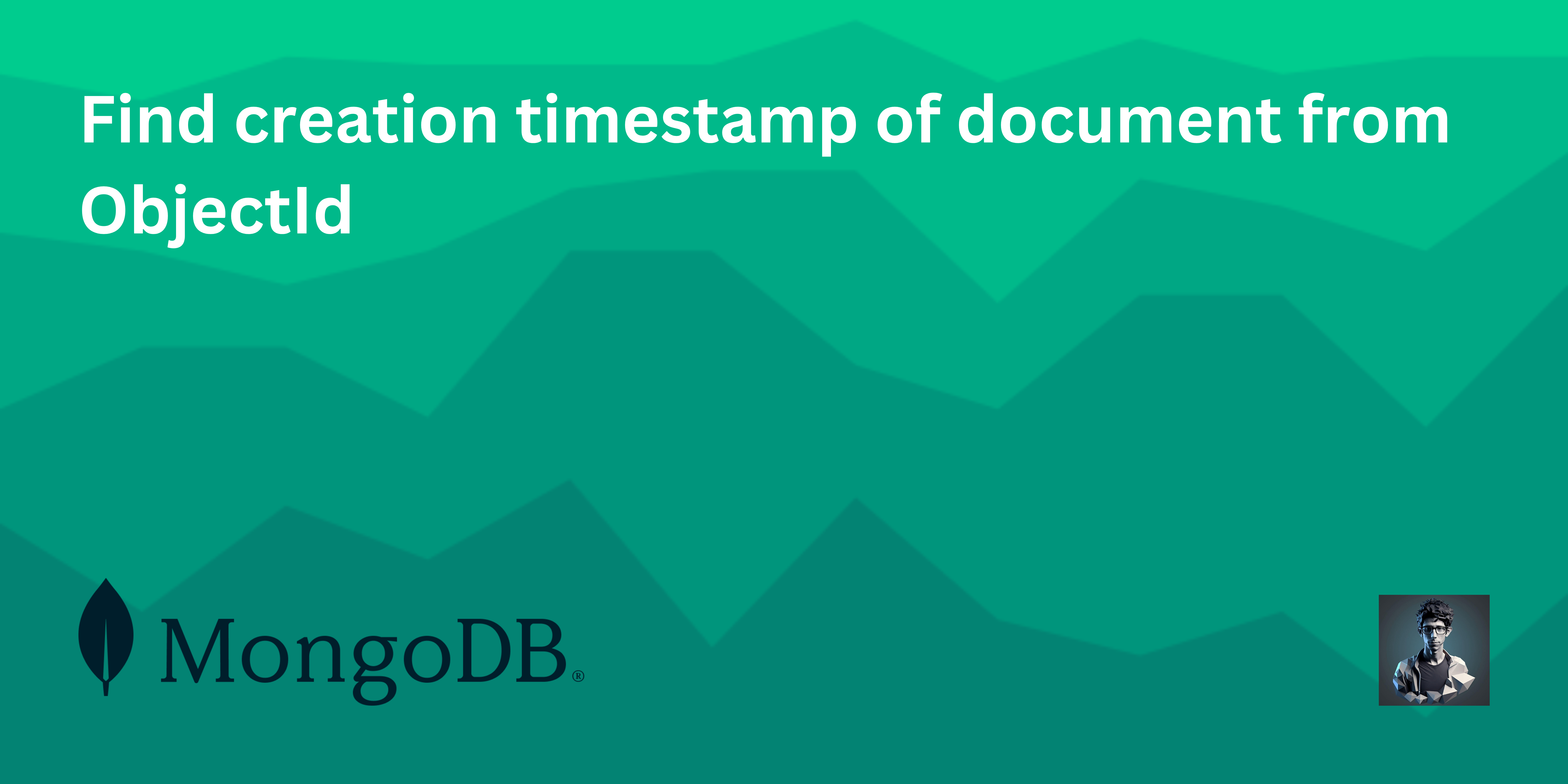
Find creation timestamp of document from ObjectId
We have below collection named student, there is no field for creation time of document. But we have to find out creation timestamp of all documents.
Solve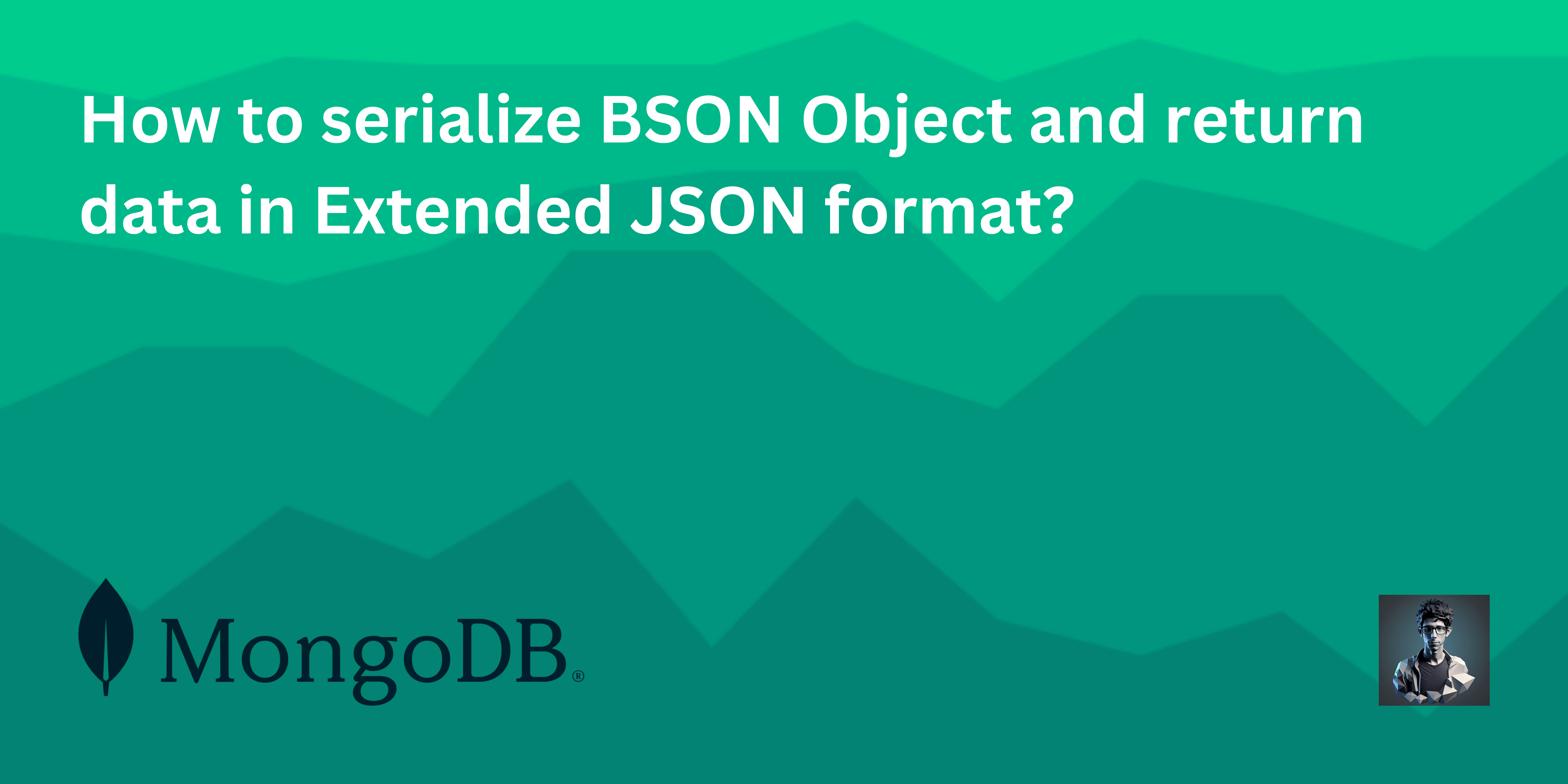
How to serialize BSON Object and return data in Extended JSON format?
We have following collection movies, write query to serialize BSON object to extended JSON for document where movie title is 'The Dark Knight'
Solve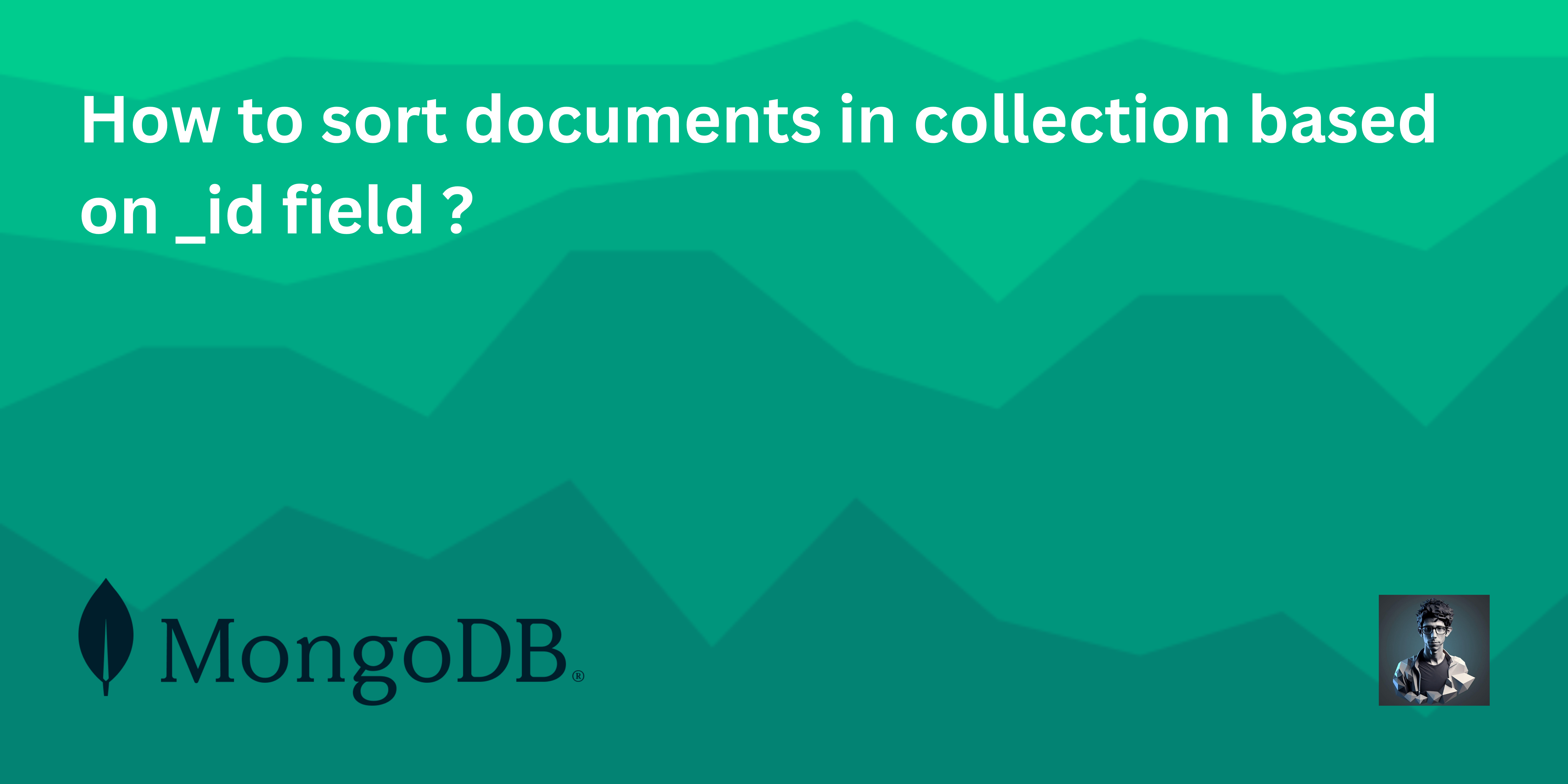
How to sort documents in collection based on _id field ?
Write mongoDB query for sorting documents in descending and asceding order based on _id field.
Solve