What is $type operator in MongoDB ? and how to use it?
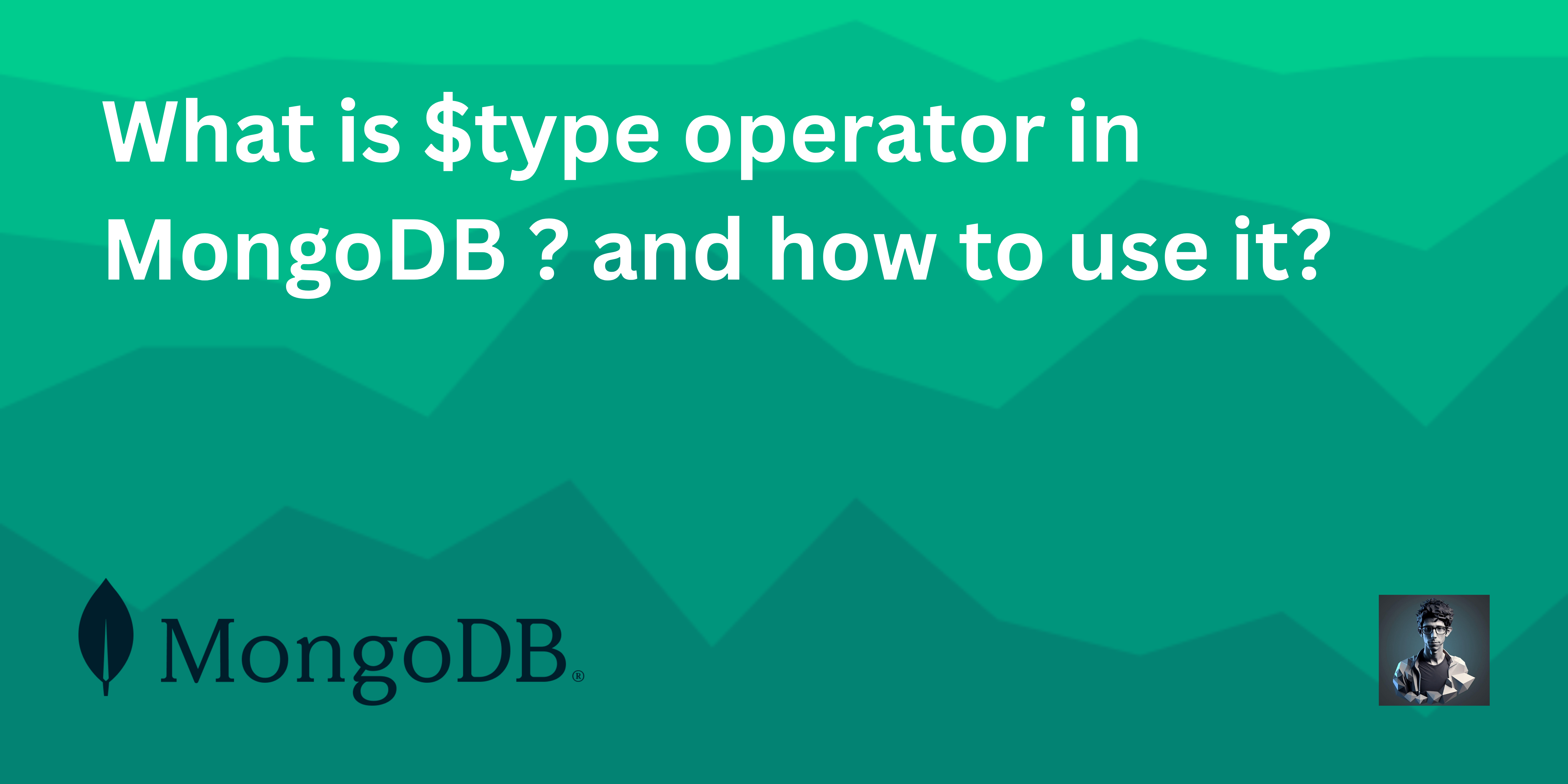
If you want to query to find the field in document where its type is something like string, int etc. then you should use $type operator.
Syntax of $type
{$type: <BSON Data Type>}
Let take one example, consider we have collection student, and let's insert one record inside it
db.students.insertOne({
"name": "Joe Rogan",
"age": 25,
"is_student": true,
"birth_date": ISODate("1999-03-15T00:00:00Z")
})
During insertion of document we have not provided _id field so mongodb will automatically create one unique Object Id for this document
Now we have to find documents in collection where name field has string BSON data type
db.students.find({"name":{$type:"string"}})
The result returned by above query is
{
_id: ObjectId('602e693f7e8df9001191c1a1'),
name: 'John Doe',
age: 25,
is_student: true,
birth_date: ISODate('1999-03-15T00:00:00.000Z')
}
Let's see what are different BSON type that we can use with $type
BSON Type | alias | Number |
---|---|---|
Double | double | 1 |
String | string | 2 |
Object | object | 3 |
Array | array | 4 |
Binary Data | binData | 5 |
Undefined | undefined | 6 |
ObjectId | objectId | 7 |
Boolean | bool | 8 |
Date | date | 9 |
Null | null | 10 |
Regular Expression | regex | 11 |
DB Pointer | dbPointer | 12 |
JavaScript | javascript | 13 |
Symbol | symbol | 14 |
32-bit Integer | int | 16 |
Timestamp | timestamp | 17 |
64-bit integer | long | 18 |
Decimal128 | decimal | 19 |
Min Key | minKey | -1 |
Max Key | maxKey | 127 |
In query we have to use one of aliases i.e string or number for given BSON type
$type operator support both string and number alias for BSON data type.
Let's just write above query again with number alias for string
db.students.find({"name":{$type:2}})
Examples
For all examples we are using collection named dataTypes which
contain fields for every bson data types.
Also for each query we will use projection document to project
only required fields instead of all fields
db.dataTypes.insertOne({
"String": "Codediggy",
"32-bit integer": NumberInt(1),
"64-bit integer": NumberLong(1000),
"decimal128": NumberDecimal(1.4),
"double": Double(1.5),
"boolean": true,
"object": { "field": "test" },
"regular expression": /^c/,
"javascript": function square(num) {return num * num;},
"timestamp": Timestamp(),
"date": Date(),
"min Key": MinKey(),
"max Key": MaxKey(),
"array": [],
"Null": null
})
1) Double
Syntax
db.collection.find({"fieldName":{$type:"double"}})
or
db.collection.find({"fieldName":{$type:1}})
/* replace fieldName with actual field name from document and collection with collection name */
Query for dataTypes Collection
db.dataTypes.find({"double":{$type:"double"}},{"double":1})
In above query we used { "double" : 1 } to project only field named double.
Result:
[ { _id: ObjectId('6623311e6548c947e716c9b6'), double: 1.5 } ]
2) String
Syntax
db.collection.find({"fieldName":{$type:"string"}})
or
db.collection.find({"fieldName":{$type:2}})
/* replace fieldName with actual field name from document and collection with collection name */
Query for dataTypes Collection
db.dataTypes.find({"String":{$type:"string"}},{"String":1})
In above query we used { "String" : 1 } to project only field named String.
Result:
[ { _id: ObjectId('6623311e6548c947e716c9b6'), String: 'codediggy' } ]
3) Object
Syntax
db.collection.find({"fieldName":{$type:"object"}})
or
db.collection.find({"fieldName":{$type:3}})
/* replace fieldName with actual field name from document and collection with collection name */
Query for dataTypes Collection
db.dataTypes.find({"object":{$type:"object"}},{"object":1})
In above query we used { "object" : 1 } to project only field named object.
Result:
[
{
_id: ObjectId('6623311e6548c947e716c9b6'),
object: { field: 'test' }
}
]
4) 32-bit Integer
Syntax
db.collection.find({"fieldName":{$type:"int"}})
or
db.collection.find({"fieldName":{$type:16}})
/* replace fieldName with the actual field name from the document and collection with the collection name */
Query for dataTypes Collection
db.dataTypes.find({"32-bit integer":{$type:"int"}},{"32-bit integer":1})
In the above query, we used { "32-bit integer" : 1 } to project only the field named "32-bit integer".
Result:
[ { _id: ObjectId('6623311e6548c947e716c9b6'), "32-bit integer": 1 } ]
5) 64-bit Integer
Syntax
db.collection.find({"fieldName":{$type:"long"}})
or
db.collection.find({"fieldName":{$type:18}})
/* replace fieldName with the actual field name from the document and collection with the collection name */
Query for dataTypes Collection
db.dataTypes.find({"64-bit integer":{$type:"long"}},{"64-bit integer":1})
In the above query, we used { "64-bit integer" : 1 } to project only the field named "64-bit integer".
Result:
[
{
_id: ObjectId('6623311e6548c947e716c9b6'),
'64-bit integer': Long('1000')
}
]
6) Decimal128
Syntax
db.collection.find({"fieldName":{$type:"decimal"}})
or
db.collection.find({"fieldName":{$type:19}})
/* replace fieldName with the actual field name from the document and collection with the collection name */
Query for dataTypes Collection
db.dataTypes.find({"decimal128":{$type:"decimal"}},{"decimal128":1})
In the above query, we used { "decimal128" : 1 } to project only the field named "decimal128".
Result:
[
{
_id: ObjectId('6623311e6548c947e716c9b6'),
decimal128: Decimal128('1.4')
}
]
7) Boolean
Syntax
db.collection.find({"fieldName":{$type:"bool"}})
or
db.collection.find({"fieldName":{$type:8}})
/* replace fieldName with the actual field name from the document and collection with the collection name */
Query for dataTypes Collection
db.dataTypes.find({"boolean":{$type:"bool"}},{"boolean":1})
In the above query, we used { "boolean" : 1 } to project only the field named "boolean".
Result:
[ { _id: ObjectId('6623311e6548c947e716c9b6'), boolean: true } ]
8) Regular Expression
Syntax
db.collection.find({"fieldName":{$type:"regex"}})
or
db.collection.find({"fieldName":{$type:11}})
/* replace fieldName with the actual field name from the document and collection with the collection name */
Query for dataTypes Collection
db.dataTypes.find({"regular expression":{$type:"regex"}},{"regular expression":1})
In the above query, we used { "regular expression" : 1 } to project only the field named "regular expression".
Result:
[
{
_id: ObjectId('6623311e6548c947e716c9b6'),
'regular expression': /^c/
}
]
9) JavaScript
Syntax
db.collection.find({"fieldName":{$type:"javascript"}})
or
db.collection.find({"fieldName":{$type:13}})
/* replace fieldName with the actual field name from the document and collection with the collection name */
Query for dataTypes Collection
db.dataTypes.find({"javascript":{$type:"javascript"}},{"javascript":1})
In the above query, we used { "javascript" : 1 } to project only the field named "javascript".
Result:
[
{
_id: ObjectId('6623311x e6548c947e716c9b6'),
javascript: Code('function square(num){return num*num;}')
}
]