Commands that you should know while using Git
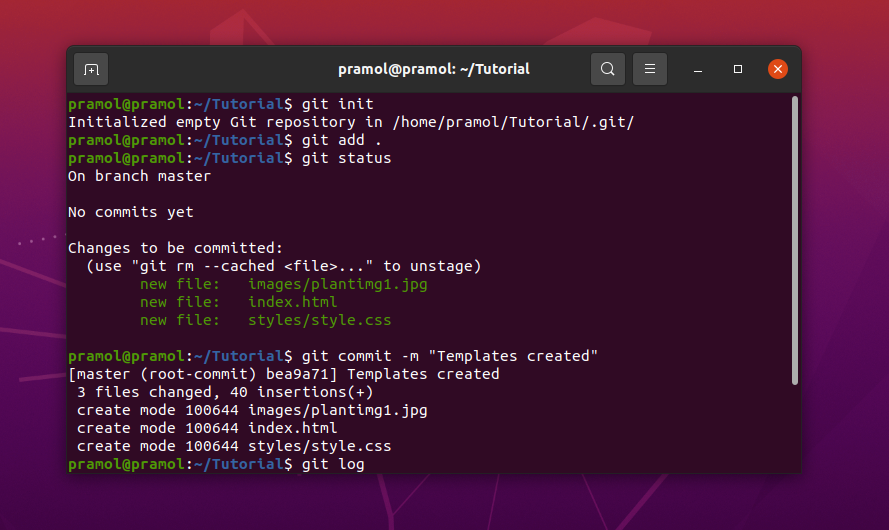
It is important to know Git commands if you want to use Git for version control in your projects. Git is a widely used distributed version control system that allows you to track changes in your code and collaborate with others.
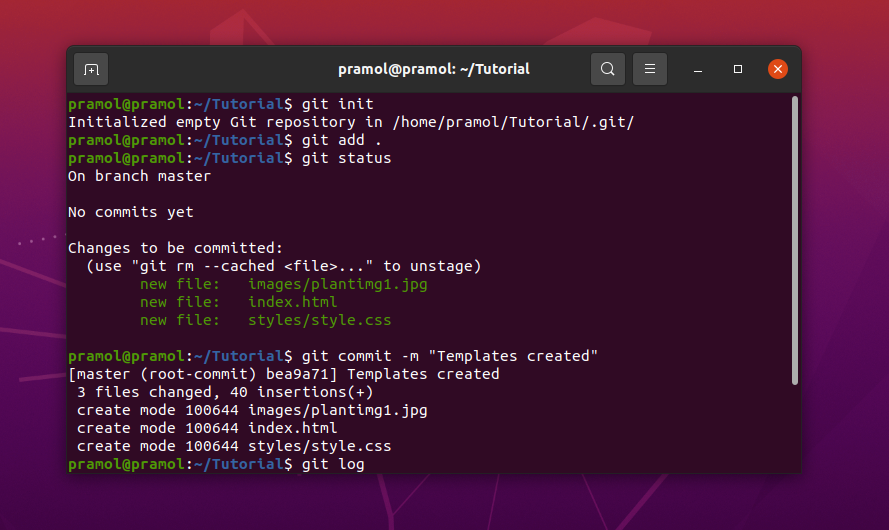
Git is a distributed version control system that allows developers to track changes to code and collaborate on software development projects. With Git, developers can make changes to their code, test those changes, and share their work with others without worrying about losing their work or overwriting someone else's work.
How Git help in Project ?
Git is useful when creating large projects because it provides several benefits that make collaboration easier and more efficient. These benefits include:
- Version control: Git keeps track of all changes made to code over time, allowing developers to revert to earlier versions of the code if necessary.
- Navigation: Git Graph provides an easy-to-use interface for navigating through a repository's history. Developers can quickly find specific commits, branches, and tags, and view detailed information about each one.
- Collaboration: Git Graph can help developers to collaborate more effectively by providing a clear overview of the repository's history. This makes it easier to communicate about changes and to identify any conflicts that may arise.
- Workflow management: Git Graph can also help developers to manage their workflow more effectively. It provides tools for creating and merging branches, rebasing commits, and resolving conflicts, all within a visual interface.
- Customization: Git Graph is highly customizable, allowing developers to adjust the layout, color scheme, and other settings to suit their preferences. This can help to make the tool more accessible and intuitive for individual developers.
Basic Commands used in Git
1. git init
Usage : git init is a command in Git that initializes a new repository. It creates a new Git repository in the current directory or a directory that you specify.
This will create a new .git directory in your current directory, which is the Git repository that contains all the version control information.
Now you can start adding files and making changes to them.
git init
Example : Suppose we have two files i.e test1.txt and text2.txt in the forlder 'demo'. Now we will use git to track that folder.
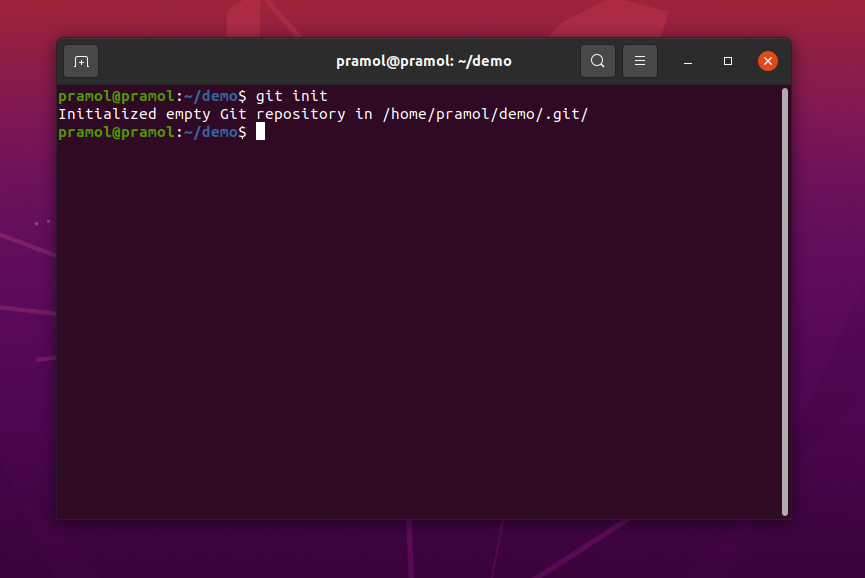
2. git status
Usage : git status is a command in Git that shows the current status of the repository, including the files that have been modified, added, deleted, or staged for commit.
To get more detailed information about the changes, use the git status -v or git status --verbose command. This will show you the exact lines that have been added or deleted.
git status
Example : We have created two files. So they are in working directory. They are untracked files we can see it by using git status
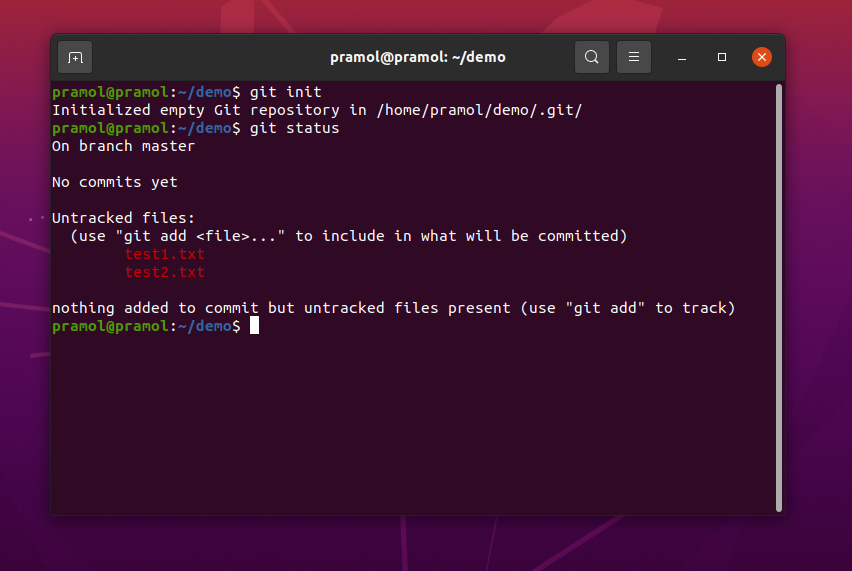
If you use git status without initializing git repository then it will give error.
3. git add
Usage : git add file_name is a command in Git that adds changes to the staging area in preparation for a commit.
To stage changes for commit, use the git add file_name command. This will add the specified file or files to the staging area.
To add multiple files to the staging area, use git add file_name1 file_name2 file_name3
git add file_name
Example : Now lets add file1.txt to the stagging area.
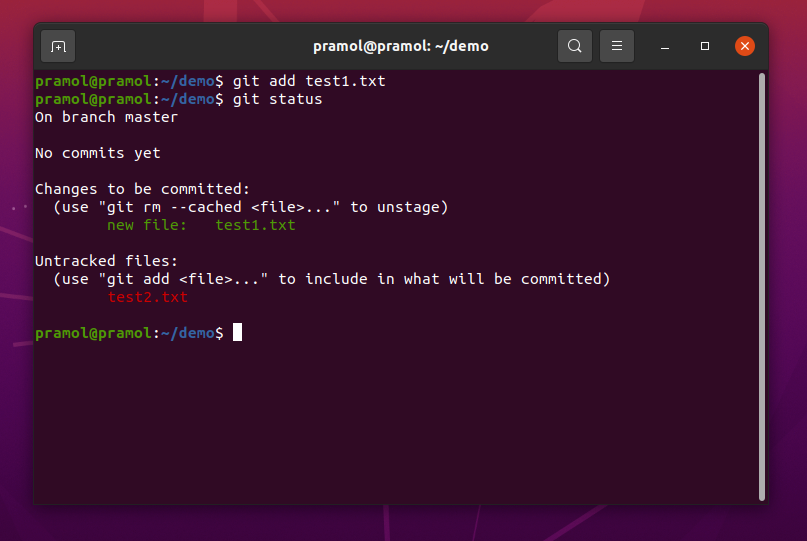
Now if we use git status then we can see the test1.txt file moved to the stagging area
4. git commit
Usage : git commit is an important command in Git that allows you to create a new commit with the changes that have been staged. It's important to write clear and concise commit messages that describe the changes you have made so that others can easily understand what has been changed.
git commit command move files to git repository from stagging area. Commit is used with option -m which help to describe why commit done
git commit -m "message"
Example : Now lets add commit changes made in file test1.txt
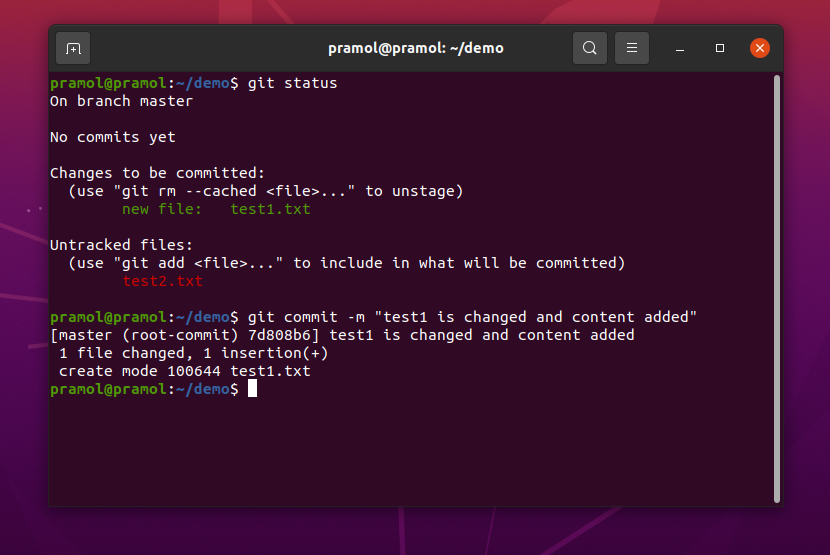
5. git log
Usage : git log is a command in Git that displays a list of commits in reverse chronological order (i.e., the most recent commit appears first).
To limit the number of commits displayed, use the git log -n number command. For example, git log -n 5 will display the five most recent commits.
To view the commit history of a specific branch, use the git log branch_name command. For example, git log feature-branch will display the commit history of the feature-branch.
git log
Example : Now If we run above command then we can result shown in below image
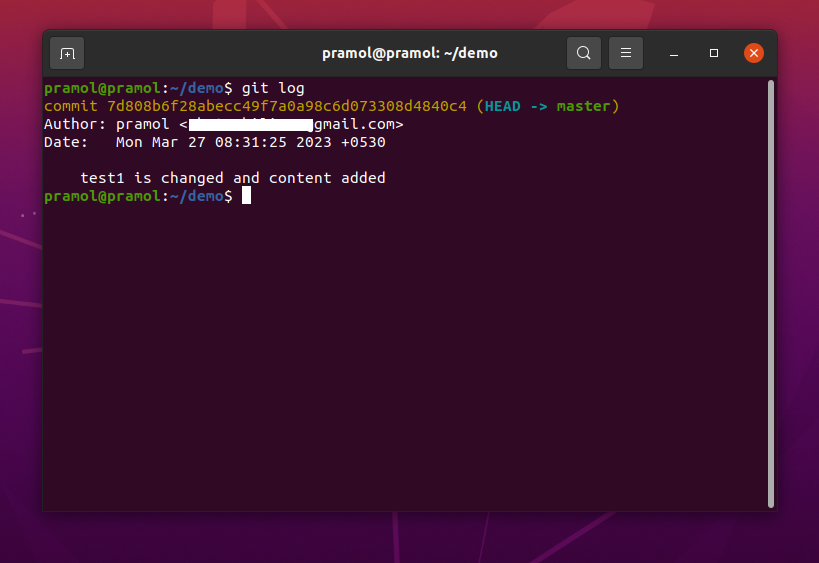
6. git branch
Usage : git branch is a command in Git that allows you to manage branches in a repository.
To view a list of branches in the repository, use the git branch command. This will display a list of all branches, with the current branch highlighted with an asterisk.
To create a new branch, use the git branch new_branch_name command. For example, git branch feature-branch will create a new branch called "feature-branch" based on the current branch.
To switch between branches, use the git checkout branch command. For example, git checkout feature-branch will switch to the "feature-branch" branch.
To create a new branch and switch to it in a single command, use the git checkout -b new_branch_name command. For example, git checkout -b feature-branch will create a new branch called "feature-branch" and switch to it.
To rename a branch, use the git branch -m old_branch_name new_branch_name command. For example, git branch -m feature-branch new-feature-branch will rename the "feature-branch" branch to "new-feature-branch".
To delete a branch, use the git branch -d branch_name command. For example, git branch -d feature-branch will delete the "feature-branch" branch.
git branch
Example : Now If we run above commands then we can result shown in below image
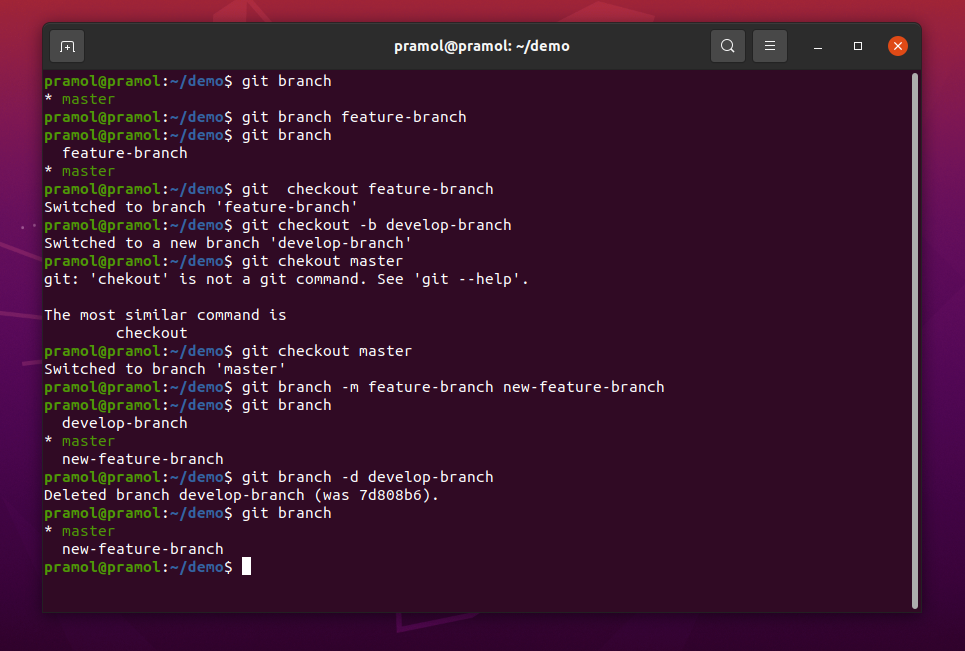
7. git clone
Usage : git clone is a command in Git that allows you to create a copy of a repository on your local machine.
To clone a repository, use the git clone repository_URL command. For example, git clone https://github.com/user/repo.git will clone the repository from GitHub to your local machine.
To clone a specific branch of a repository, use the git clone -b branch_name repository_URL command. For example, git clone -b feature-branch https://github.com/user/repo.git will clone the "feature-branch" branch of the repository to your local machine.
git clone
Example : Now clone the repository from url https://github.com/pramol-bhosale/weather-webapp.git
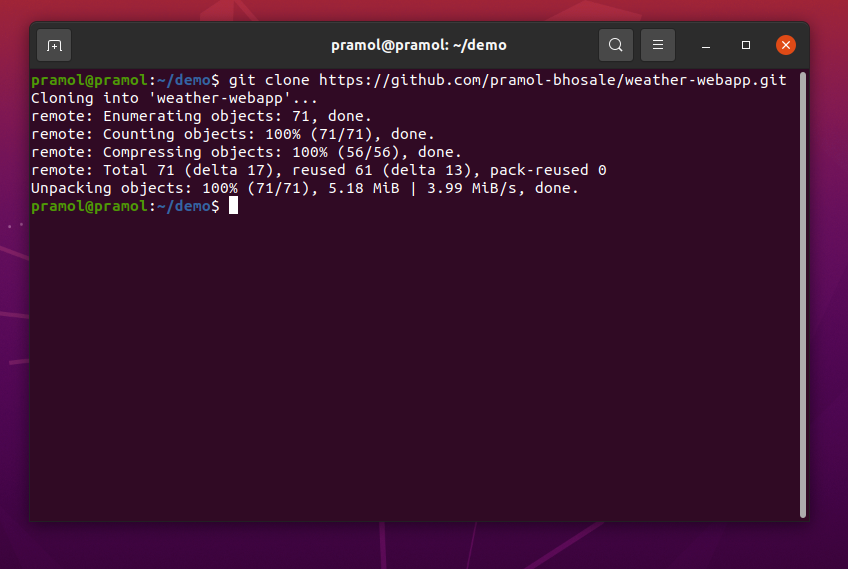
8. git push
Usage : git push is a command in Git that allows you to upload local changes to a remote repository.
To push changes to the current branch of a remote repository, use the git push command. For example, git push origin will push changes to the "origin" remote repository.
To push changes to a specific branch of a remote repository, use the git push remote branch command. For example, git push origin feature-branch will push changes to the "feature-branch" branch of the "origin" remote repository.
To push changes to a new branch of a remote repository, use the git push remote local_branch remote_branch command. For example, git push origin my-local-branch:new-remote-branch will push changes from "my-local-branch" to a new branch called "new-remote-branch" on the "origin" remote repository.
9. git pull
Usage : git pull is a command in Git that allows you to update your local repository with changes from a remote repository.
To pull changes from the remote repository into the current branch of your local repository, use the git pull command. For example, git pull origin will fetch and merge changes from the "origin" remote repository into the current branch of your local repository
To pull changes into a specific branch of your local repository, use the git pull remote branch command. For example, git pull origin feature-branch will fetch and merge changes from the "feature-branch" branch of the "origin" remote repository into the current branch of your local repository.
To pull changes from the remote repository without automatically merging them into your local branch, use the git pull --no-commit remote branch command. This can be useful if you want to review the changes before merging them.
10. git remote add
git remote add
Usage : git remote add is a command in Git that allows you to add a remote repository to your local repository
To add a remote repository to your local repository, use the git remote add name url command. For example, git remote add origin https://github.com/user/repo.git will add a remote repository called "origin" with the URL "https://github.com/user/repo.git".
You can add multiple remote repositories to your local
repository by using different names for each repository. For
example, you could add a second remote repository called
"upstream" using the command
git remote add upstream https://github.com/otheruser/repo.git
To verify the remote repositories associated with your local repository, use the git remote -v command. This will list the remote repositories along with their URLs.
To change the URL of a remote repository, use the git remote set-url name new_url command. For example, git remote set-url origin https://github.com/user/new-repo.git will change the URL of the "origin" remote repository to "https://github.com/user/new-repo.git".
To remove a remote repository from your local repository, use the git remote remove name command. For example,framework git remote remove upstream will remove the "upstream" remote repository.